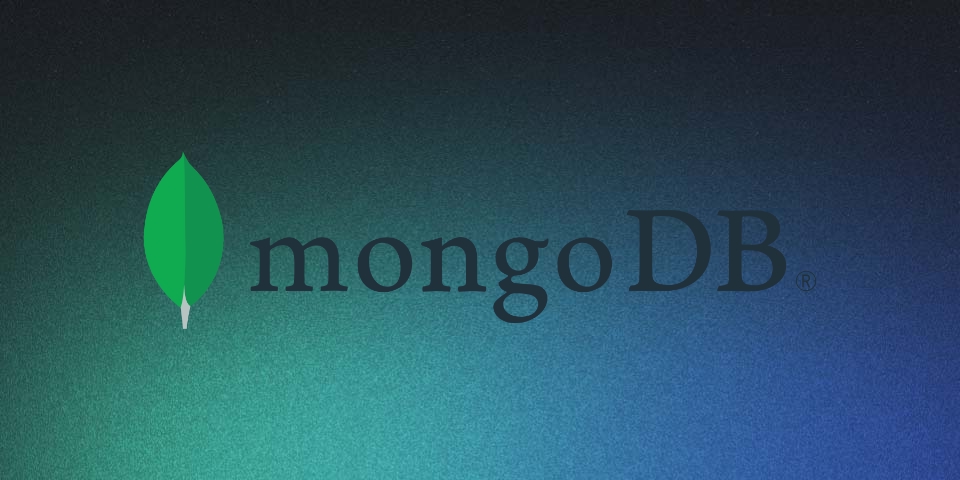
Why I’m Moving Away from MongoDB (and What You Need to Know)
MongoDB’s document model and schemaless design have made it a popular choice for rapid prototyping. In my early projects, the “it’s so easy” mantra was a huge selling point. However, as my applications grew, so did the drawbacks—especially when it came to handling relationships.
The Lure of “Easy”
MongoDB’s flexible schema allowed me to get up and running quickly without the overhead of defining tables and relationships. It felt liberating—until the projects scaled. When you don’t enforce data types or relationships at the database level, inconsistencies can creep in. For example, using Mongoose you can simulate relationships, but these are purely application-level constructs. There’s little help catching type mismatches during development or ensuring that when you delete a record the related documents are handled appropriately.
The Pitfalls I Discovered
Over time I encountered several issues:
- Data Inconsistencies: Without a strict schema, parts of the application started writing data in different formats. What began as an advantage turned into a maintenance headache.
- Painful Migrations: When evolving the data model, I had to rely on manual or ad hoc solutions for schema migrations. In contrast, relational databases offer mature migration tools (e.g., Liquibase, Prisma Migrate) that ensure changes are reversible and reliable.
- Lack of Native Relationship Support: This is a critical issue. In MongoDB, if you delete a document (like a user), there’s nothing stopping you from leaving orphaned references in other collections. In SQL databases, you can define foreign key constraints with actions like
ON DELETE CASCADE
—and have the database enforce referential integrity for you.
The Missing Piece: Native Relationship Management
One of the major drawbacks of MongoDB is its lack of native support for relationships. In a true relational database, foreign key constraints enforce integrity at the database level. When you delete a record, the database can automatically cascade the deletion to related records using options such as ON DELETE CASCADE
or ON DELETE SET NULL
. Imagine deleting a user and receiving an error because that user has posts referencing them. In MongoDB, however, if you delete a user, nothing stops you from having “dangling references” in other collections.
This gap forces developers to implement cascading deletes manually (often using Mongoose middleware). For example, you might set up a pre-remove hook on a user model to query and delete all posts that reference that user. Not only does this add extra code, but it also brings performance implications:
- Additional Database Round-Trips: Manual cascade deletes typically involve multiple queries and must be wrapped in transactions to ensure consistency.
- False Sense of Security: If you rely solely on middleware, you might forget to account for cases when a document is deleted by other means (like
deleteOne()
), leaving orphaned data behind.
Fact-Checking My Experience
Many developers have encountered similar challenges. Discussions on GitHub and articles on migration blockers point out that:
- Schema Evolution Can Be Painful: Migrating a MongoDB schema often requires custom scripts and additional safeguards that are built into mature SQL migration frameworks.
- Type Safety Issues Abound: Mongoose and similar libraries can’t fully enforce type safety at the database level—leading to potential runtime errors that wouldn’t happen with a strongly typed relational database.
- Performance Hits: The manual effort of ensuring referential integrity in MongoDB (by maintaining cascade deletes in code) adds extra overhead and complexity, which can hurt performance, especially under heavy load.
Why PostgreSQL (with Prisma) Is a Better Fit
Switching to PostgreSQL—paired with an ORM like Prisma—offers several advantages:
- Robust Type Safety: PostgreSQL enforces a strict schema and data types, catching issues at compile time (especially in TypeScript projects).
- Mature Migration Tools: With tools like Prisma Migrate, you can make schema changes in a predictable, reversible manner, integrated seamlessly into your CI/CD pipelines.
- Native Referential Integrity: Foreign key constraints and cascading actions (e.g.,
ON DELETE CASCADE
) ensure that you cannot accidentally leave orphaned records behind. - ACID Transactions: PostgreSQL’s commitment to ACID compliance guarantees data consistency, even in the face of complex transactions and migrations.
In Conclusion
MongoDB is great for getting projects off the ground quickly. However, its lack of native relationship management—especially the absence of foreign key constraints and cascading deletes—can lead to data integrity issues as your application scales. I’ve seen firsthand how the flexibility of MongoDB can turn into a long-term liability.
For production-grade applications where data integrity and maintainability are paramount, PostgreSQL (backed by an ORM like Prisma) offers a more disciplined approach. It provides native tools to enforce relationships, catch inconsistencies early, and ensure that your data remains robust as your project grows.
Happy coding!